Rows
Row Pinning
Row pinning is driven by the Row Data Source
currently present in the grid. There are two types of row data sources,
client
and controlled
, each with its way of specifying how many
rows should be pinned to the top and bottom of the grid.
Specifying Row Pinning
Client Source
For the full description of the client row data source, see Client Data Source.
const clientDataSource = {
kind: "client",
data: rowData,
topData: [
["AAPL", 147.87, 149.64, 89000000, 2.4e12, 28.7, 0.0065, 157.26],
["MSFT", 305.41, 308.3, 55000000, 2.3e12, 35.4, 0.0087, 349.67],
],
bottomData: [
["JPM", 130.42, 132.45, 12000000, 410.5e9, 10.1, 0.0284, 165.3],
["BAC", 37.65, 38.0, 9800000, 307.2e9, 11.2, 0.0221, 45.88],
],
};
When using a client data source, Graphite Grid can determine the number of rows to pin at the top or bottom based on the provided data.
Controlled Data Source
For the full description of the controlled row data source, see Controlled Data Source.
const controlledDataSource = {
kind: "controlled",
getRowTopCount() {
return 2;
},
getRowBottomCount() {
return 2;
},
// other properties
};
When using a controlled data source, Graphite Grid will call getRowTopCount
and
getRowBottomCount
to determine the number of rows to pin at the top and bottom.
Basic Example
export function RowPinned() {
const grid = useGraphiteGrid({
initial: {
rowDataSource: {
kind: "client",
data: rowData,
topData: [
["AAPL", 147.87, 149.64, 89000000, 2.4e12, 28.7, 0.0065, 157.26],
["MSFT", 305.41, 308.3, 55000000, 2.3e12, 35.4, 0.0087, 349.67],
],
bottomData: [
["JPM", 130.42, 132.45, 12000000, 410.5e9, 10.1, 0.0284, 165.3],
["BAC", 37.65, 38.0, 9800000, 307.2e9, 11.2, 0.0221, 45.88],
],
},
// other props
},
});
return (
<div style={{ height: 400 }}>
<GraphiteGridDom state={grid} />
</div>
);
}
Row Indices with Pinned Rows
When using pinned rows, Graphite Grid starts counting the row indices from the first pinned row, then the unpinned rows, and finally the bottom pinned rows. For example, if there are 2 rows pinned at the top, 10 unpinned rows, and 3 rows pinned at the bottom:
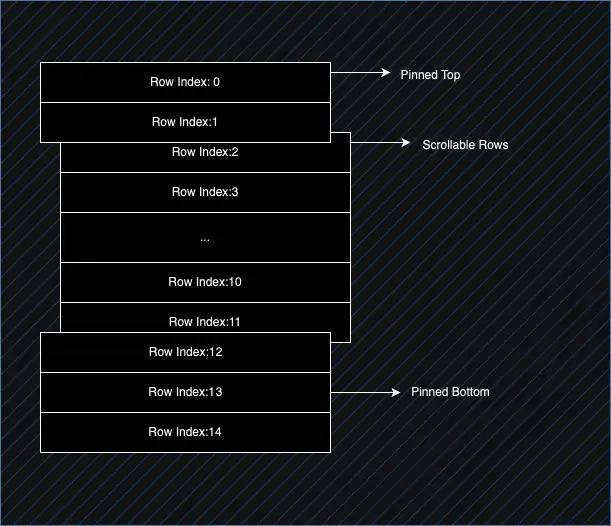
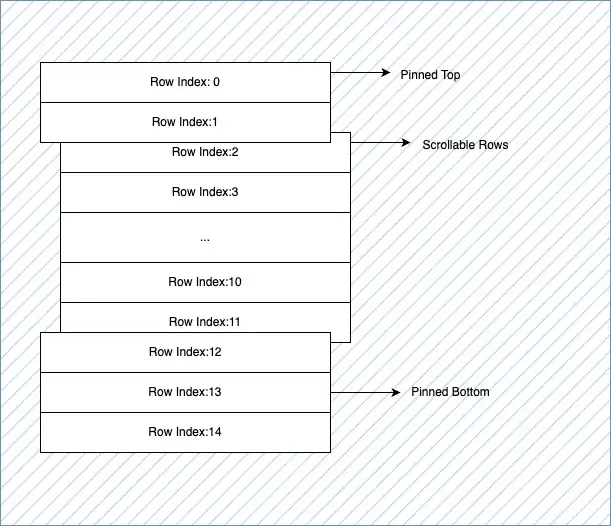